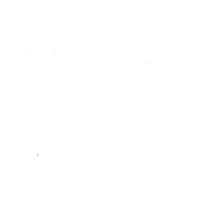
Daisy UI Code Mockup Script

Scripts used to generate HTML or JSX to be used within one of Daisy UI's code mockups. These can be seen used throughout my site.
Also allows for syntax highlighting, which you specify in a .json file named config.json into the sample directory, which store the regex and highlight colour for syntax highlighting. Two categories, keywords and other. Example ReGex are within the configs directory of the GitHub repo, but these .json files need to be renamed and moved in order to work.
Usage instructions can be found on the GitHub README, linked above.
Development + Implementation
I wanted to display the code of some of my simpler projects like scripts within my portfolio site. However, I did not want to include a screenshot of my code, since I appreciate the ability to be able to simply copy + paste code from a website, which that wouldn't allow. I was already using DaisyUI for many of the UI components, and I liked how the code blocks looked and so I chose to use it.
This is a Python script that analyzes the code in the specified file, and goes line by line converting it into a format that HTML/JSX allows. There is also syntax highlighting, but this was chosen to be ommitted on this site specifically for the sake of smaller packages being sent to the user.
import os
import sys
import re
import json
INVALID_INPUT = 'Invalid Input, Exitting...'
def find_and_replace_string(reg, line):
for (regex, edit) in reg:
words = re.findall(regex, line)
visited = {}
for word in words:
if word not in visited.keys():
visited[word] = 1
line = line.replace(word, '\"}{<span className=\"' + edit + '\">{\"' + word + '\"}</span>}{\"')
return line
def find_and_replace_word(reg, line):
temp = line.split(' ')
line = ''
for word in temp:
for (regex, edit) in reg:
if re.search(regex, word):
word = '\"}{<span className=\"' + edit + '\">{\"' + word + '\"}</span>}{\"'
break
line += word + ' '
return line
def parse_input(fp, jsx):
lines = fp.readlines()
keywords = []
other = []
strings = []
comments= []
if os.path.exists('config.json'):
with open('config.json', 'r') as cfg:
j = json.load(cfg)
keywords = j["keywords"]
other = j["other"]
strings = j["strings"]
comments = j["comments"]
if jsx:
for i in range(len(lines)):
curr = lines[i].replace('\n', '').replace('\\', '\\\\').replace('\'', '\\\'').replace('\"', '\\\"')
if strings:
curr = find_and_replace_string(strings, curr)
if other:
curr = find_and_replace_word(other, curr)
if keywords:
curr = find_and_replace_word(keywords, curr)
lines[i] = f'<pre data-prefix=\"{i + 1}\">' + '<code>{\"'+ curr + '\"}</code></pre>\n'
else:
for i in range(len(lines)):
lines[i] = f'<pre data-prefix=\"{i}\">' + '<code>'+ lines[i].replace('\n', '') + '</code></pre>\n'
output_name = 'output_jsx' if jsx else 'output_html'
with open(output_name, 'w', encoding="utf-8") as out:
out.writelines(lines)
def handle_inputs():
if len(sys.argv) > 3:
print(INVALID_INPUT)
else:
if (os.path.exists(sys.argv[1])):
with open(sys.argv[1], 'r', encoding='utf-8') as fp:
parse_input(fp, 'jsx' in sys.argv)
else:
print(INVALID_INPUT)
handle_inputs()